Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
Tags
- 자바스크립트
- DATABASE
- reactjs
- javascript
- 컴포넌트
- springboot
- jdk
- GIT
- Vue
- restapi
- JS
- EXTJS
- Spring
- table
- 보안취약점
- mssql
- 스프링
- Intellij
- 개발
- sql
- 데이터베이스
- 쿼리
- 개발공부
- React
- 리액트
- 스프링부트
- 자바
- component
- crud
- java
Archives
- Today
- Total
준준의 기록일지
[React] 컴포넌트 라이프사이클(+타이머 예제) 본문
"이 포스팅은 쿠팡 파트너스 활동의 일환으로, 이에 따른 일정액의 수수료를 제공받습니다."
라이프사이클 : 어떤 객체가 생성부터 파괴되는 시점 사이에서 자동으로 호출되는 메서드들의 집합
컴포넌트 생성과 DOM에 마운트
- 컴포넌트가 생성되고, DOM에 마운트(추가)될 때 다음과 같은 순서로 메서드가 호출된다. 이러한 과정은 딱 한번만 반복된다
- constructor(props) : 객체가 생성될 때
- componentWillMount() : 컴포넌트가 DOM에 마운트되기 직전
- render() : 컴포넌트가 렌더링될 때
- componentDidMount() : 컴포넌트가 DOM에 마운트된 직후.
컴포넌트 업데이트
- 컴포넌트의 프로퍼티가 변경되면 다음과 같은 메서드가 호출된다.
- componentWillReceiveProps(nextProps) : 컴포넌트의 프로퍼티가 변경될 때, 새로운 속성을 받았을때 호출된다.
- 이 메서드 내부에서 setState()메서드를 호출해 컴포넌트의 상태를 변경할 수 있다. 다른 업데이트 계열의 라이프사이클에서 setState()를 호출하면 업데이트 이벤트가 재귀적으로 발생하므로 setState()는 해당 메서드 내부에서만 사용하는 것이 좋다.
- shouldComponentUpdate(nextProps, nextState) : 컴포넌트의 외관을 변경해도 좋을지 판단할 때
- true or false를 반환한다.
- 성능 튜닝이 필요한 경우에 활용하면 좋다고 한다.
- componentWillUpdate() : 컴포넌트가 업데이트되기 직전
- render() : 컴포넌트가 렌더링될 때
- componentDidUpdate : 컴포넌트가 업데이트된 직후
DOM에서 언마운트
- 컴포넌트가 DOM에서 언마운트(제거)될 때 다음과 같은 메서드가 호출된다.
- componentWillUnmount()
src/StopWatch.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
|
import React, { Component } from 'react';
class Stopwatch extends Component {
constructor(props){
super(props)
this.state = {
isLive : false,
curTime : 0,
startTime : 0
}
this.timerId = 0
}
componentWillMount(){
this.timerId = setInterval(e => {this.tick()}, 1000)
}
componentWillUnmount(){
clearInterval(this.timerId)
}
tick(){
if(this.state.isLive){
this.setState({
curTime : new Date().getTime()
})
}
}
getDisp (){
const s = this.state
const delta = s.curTime - s.startTime /* 유닉스 타임으로 시간 구하기. */
const t = Math.floor(delta / 1000)
const ss = t % 60
const m = Math.floor(t / 60)
const mm = m % 60
const hh = Math.floor(mm / 60)
const z = (num) => {
const s = '00' + String(num)
return s.substr(s.length - 2, 2)
}
return <span className = 'disp'>
{z(hh)}:{z(mm)}:{z(ss)}
</span>
}
render(){
let label = 'START'
if(this.state.isLive){
label = 'STOP'
}
return (
<div>
<div>{this.getDisp()}</div>
<button onClick={(e)=>{
if(this.state.isLive){
//STOP 클릭
this.setState({
isLive : false
})
} else{
//START 클릭
const v = new Date().getTime()
this.setState({
curTime : v,
startTime : v,
isLive : true
})
}
}}>{label}</button>
</div>
);
}
}
export default Stopwatch;
|
cs |
이후 App.js return (<Stopwatch />) 로 컴포넌트를 추가한 뒤
npm start로 서버를 구동하면 동작하는 것을 확인할 수 있다.
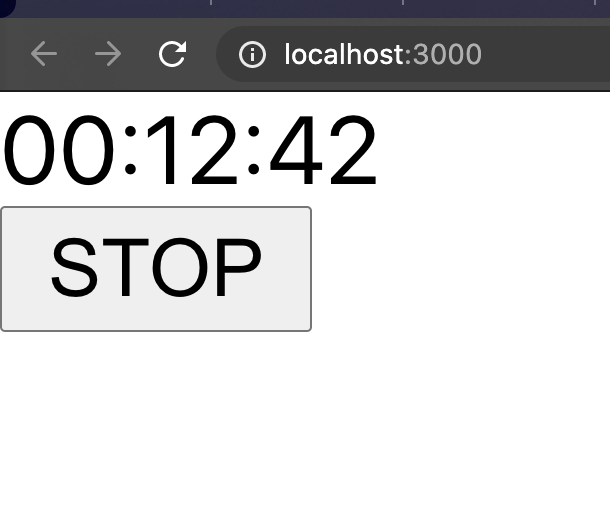
출처 : 모던 자바스크립트 개발자를 위한 리액트 프로그래밍 3장
'ReactJS' 카테고리의 다른 글
[react] React Fast REfresh 2020.01 (0) | 2021.01.12 |
---|---|
[React] SPA - 웹 서버, 리액트 (0) | 2021.01.08 |
[React] JSON 형태로 입력 데이터 출력 (0) | 2020.12.29 |
[ReactJS] React Component (0) | 2020.12.23 |
[nodJs] npm 기본 (0) | 2020.12.15 |